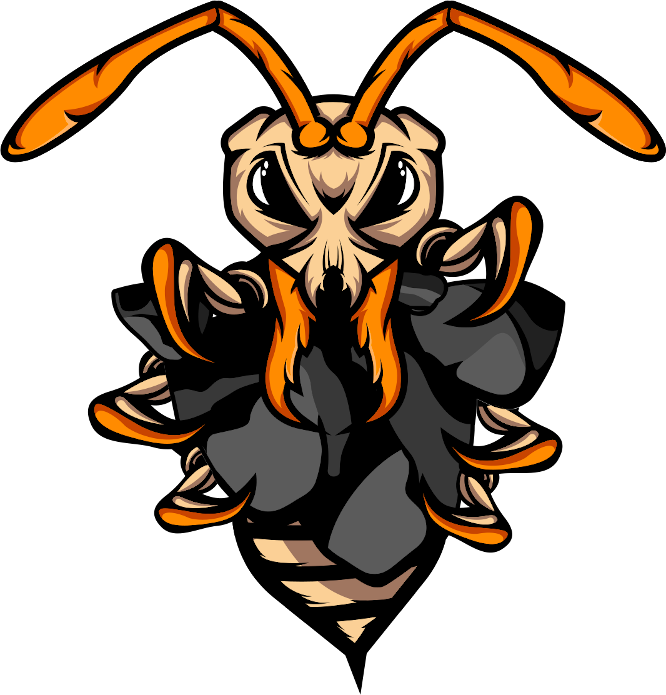
Nift has its own in-built scripting language
Contents
f++ interpreter
In the interpreter mode the prompt will just tell you which language you are using. If you would like the prompt to also display the present working directory (up to using half the width of the console) you can switch to the shell mode using
You can switch to one of the other languages available in Nift's interpreter using
f++ shell
In the shell mode the prompt will tell you which language you are using and the present working directory (up to using half the width of the console). If you would like the prompt to just display the language you can switch to the interpreter mode using
You can switch to one of the other languages available in Nift using
Running f++ scripts
If you have an
nsm run path/script-name.f nift run path/script-name.f
If the script has a different extension, say
nsm run -f++ path/script-name.ext nift run -f++ path/script-name.ext
If you want to run a normal file, eg.
#!/usr/bin/env nift
There's information about "run commands" scripts, eg.
Automatic shell startup
On Unix based platforms (eg. probably all posix compliant Linux distributions, OSX, FreeBSD, Gentoo etc.), you can create an application launcher with
From
#!/usr/bin/env xdg-open [Desktop Entry] Version=1.0 Type=Application Terminal=true Exec=x-terminal-emulator -e nift sh Name=Terminal Comment=flashell shortcut Icon=<path-to-icon>
I often find it easier to just use the properties of the file through the gui to acccess the filesystem for linking to the icon file, typically from the desktop. Below is an icon people might like to use with their short-cut(s):
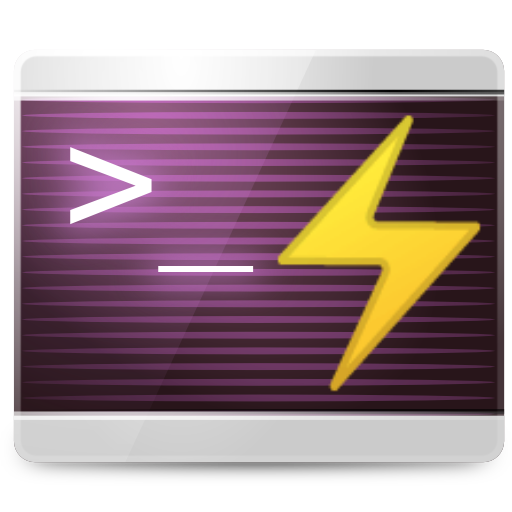
If you prefer a more native feel without the icon being hijacked, the default icon used for
and the default icon, or at least one similar to it, used for
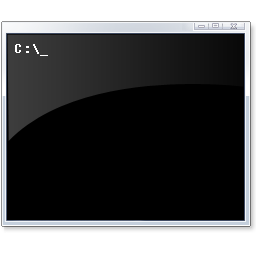
You can start a session of eg. bash shell by simply typing
On Windows one approach is to create a shortcut to
f++ from n++
You can run
@f++(/* single line of f++ code */)
@f++ { // block of f++ code }
Nift functions
All of Nift's hard-coded functions (including functions for defining variables, functions and structs) are available in your
funcName{options}(params)
Examples
There are some basic solutions to Project Euler problems available here.
The following script will create and delete 100k files on your machine:
#!/usr/bin/env nift string params = "file0.txt"; for(int i=1; i<100000; i+=1) $`params += ', file$[i].txt'`; poke($[params]); rmv($[params]);
The following script uses more
#!/usr/bin/env nift string params = "file0.txt"; int i; exprtk { for(i:=1; i<100000; i+=1) { params += ', file' + nsm_tostring('i') + '.txt'; } } poke($[params]); rmv($[params]);
Note: do not leave the directory you are running the script from open on your machine, run it from a terminal. The following bash script will do the same but much slower, feel free to provide a similar windows script in powershell or a batch file:
#!/usr/bin/env bash COUNTER=0 while [ $COUNTER -lt 100000 ]; do let COUNTER=COUNTER+1 touch file${COUNTER}.txt rm file${COUNTER}.txt done